Classical Harmonic Oscillator#
What You Need to Know
Equation of Motion:
Derived from Hooke’s law, \(F = -kx\), leading to the second-order differential equation \( m \ddot{x} + kx = 0 \) governing simple harmonic motion (SHM).
Solution to the Oscillator:
The general solution is \( x(t) = A \cos(\omega t + \phi) \), with \( \omega = \sqrt{k/m} \). The amplitude \( A \) and phase \( \phi \) depend on initial conditions.
Energy in Oscillation:
Kinetic energy, \( T = \frac{1}{2} m v^2 \), and potential energy, \( U = \frac{1}{2} k x^2 \), exchange throughout the motion, while total energy remains constant.
Oscillation Parameters:
Key parameters include angular frequency \( \omega \), frequency \( f = \frac{\omega}{2\pi} \), and period \( T = \frac{2\pi}{\omega} \).
Damped and Driven Oscillations:
Discuss damping (energy dissipation) and resonance in driven systems, highlighting underdamped, overdamped, and critically damped cases.
Examples and Applications:
Includes mass-spring systems, pendulums (small angles), and LC circuits. Emphasizes the harmonic oscillator’s role in describing oscillations across different physical systems.
Bead, spring and a wall.#
The classical harmonic oscillator is a system of bead attached to a wall with a spring.
When bead is displaced from its equilibrium or resting position \(r_0\) to some point \(r\), experiences a restoring force \(F\) proportional to the displacement \(x=r-r_0\):
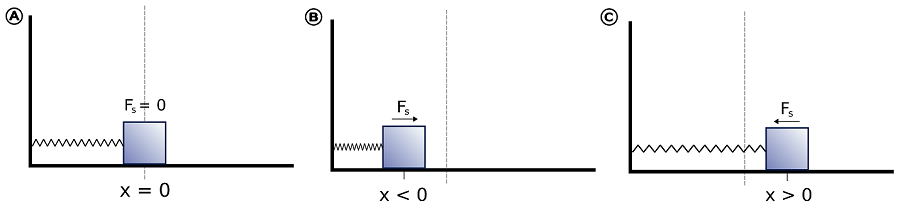
Fig. 60 Illustration of harmonic motion governed by Hook’s law. Any deviation from equilibrium (resting) position is met with restoring force. In the absence of friction the bead keeps oscillating around equilibrium position.#
This is Hooke’s law, where minus sign indicates that the direction of force is always towards restoring equilibrium location.
The constant k characterizes stiffness of the spring and is called spring constant.
Solving harmonic oscillator problem#
The classical equation of motion for a one-dimensional simple harmonic oscillator with a particle of mass m attached to a spring having spring constant k generates mechanical waves.
The intorduced constant \(\omega\) will be seen as the frequency oscillations.Note that frequency is inversly porportional to mass (heavier objects with same spring constant oscillate rapdily around equilibrium) and proprotional to spring constant (stiffer springs increase oscillations around equilibrium for same mass)
The differneital equation is a simple second order, linear ODE which can be solved by a standard trick of pluggin exponential \(x(t)=e^{\alpha t}\) and convertin the problem to algebraic equation. The solution is
The two constnants are: \(A\) the amplitude of oscillations and \(\phi\) is constant specifying the initial position of the bead.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
# Constants
A = 1 # Amplitude
phi = 0 # Phase (set to 0 for simplicity)
t = np.linspace(0, 4, 1000) # Time array
# Define angular frequency as a function of k and m
def angular_frequency(k, m):
return np.sqrt(k / m)
# Set a few values of k (spring constant) and m (mass)
k_values = [1, 2, 5] # Different spring constants
m_values = [1, 2, 5] # Different masses
# Create subplots
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(12, 6))
# Plot for different values of k with a fixed m
m_fixed = 1 # Fix mass for k variation
for k in k_values:
omega = angular_frequency(k, m_fixed)
x_k = A * np.sin(omega * t + phi)
ax1.plot(t, x_k, label=f'k = {k}')
ax1.set_title('Oscillation for different k values (m = 1)')
ax1.set_xlabel('Time (t)')
ax1.set_ylabel('Position (x)')
ax1.legend()
ax1.grid(True)
# Plot for different values of m with a fixed k
k_fixed = 1 # Fix spring constant for m variation
for m in m_values:
omega = angular_frequency(k_fixed, m)
x_m = A * np.sin(omega * t + phi)
ax2.plot(t, x_m, label=f'm = {m}')
ax2.set_title('Oscillation for different m values (k = 1)')
ax2.set_xlabel('Time (t)')
ax2.set_ylabel('Position (x)')
ax2.legend()
ax2.grid(True)
# Display the plots
plt.tight_layout()
plt.show()
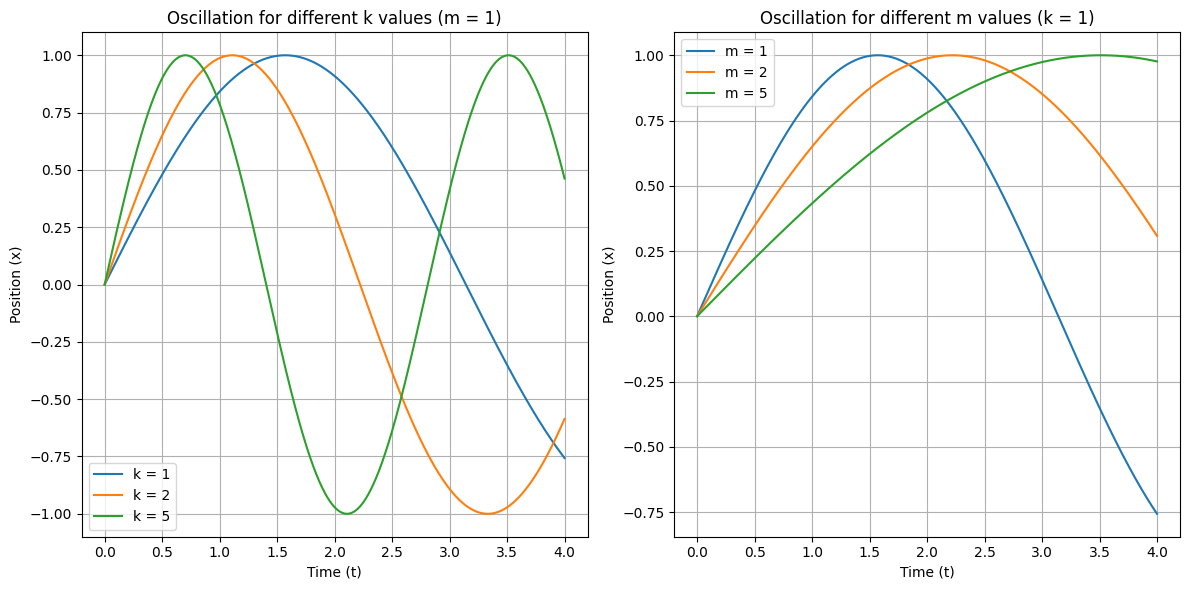
Energy of the harmonic oscillator#
In classical mechanics the force and the potential energy of a conservative system are related via the formula:
This means the steeper the potential the higher the force and minus sign indicates that force is restoring the equilibrium position. The potential energy can be obtained by integrating:
Thus the potential energy for a simple harmoncin oscillator is a parabolic function of displacement. It is convenient to set \(C=0\) and measure potential energy relative to equilibrium state \(V(x=0)=0\)
The total energy consisting of kinetic and potential enregies will be used to obtain Schordinger equation.
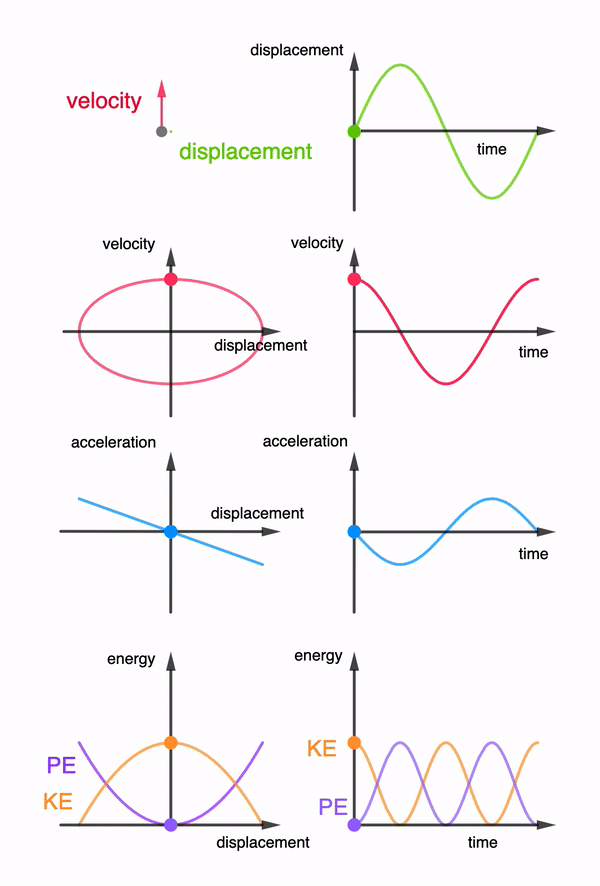
Fig. 61 The harmonic oscilator (in a vacuum) is a conservative system becasue the kinetic and potential energies keep being interconverted with no amount of total energy being dissipated into the environment. Oscillations go on forever with position \(x(t)\) velocity \(v=\dot{x}(t)\) and acceleration \(a=\ddot{x(t)}\) with same constant frequency \(\omega\) but with different amplitudes.#
Diatomic molecules and two-body problem#
Diatomic molecule is stable because the same force is acting on both ends
By expressing equations of motion in terms of the center of mass which, we find that center of mass moves freely without acceleration.
Next by taking difference between coordinates \(\ddot{x_2}=-\frac{k}{m_2}x_2\) and \(\ddot{x_1}=\frac{k}{m_1}x_1\)we expres the equations of motion in terms of relative distance
This equation looks identical to the probem of bead anchored to wall with a spring. We have thus managed to reduce the two body probelm to a one modey problem by replacing masses of bodies with a reduced mass: \(\mu=\frac{1}{m_1}+\frac{1}{m_2}=\frac{m_1 m_2}{m_1+m_2}\)
Beads and springs model of molecules#
Before discussing the harmonic oscillator approximation let us reflect on when this would be a good approximation and uner which cirumstances it will break down? For an aribtarry potential energy funciton of x we can carry out Taylor’s expansion around equilibrium bond length \(x_0\) obtaining infinitey series.
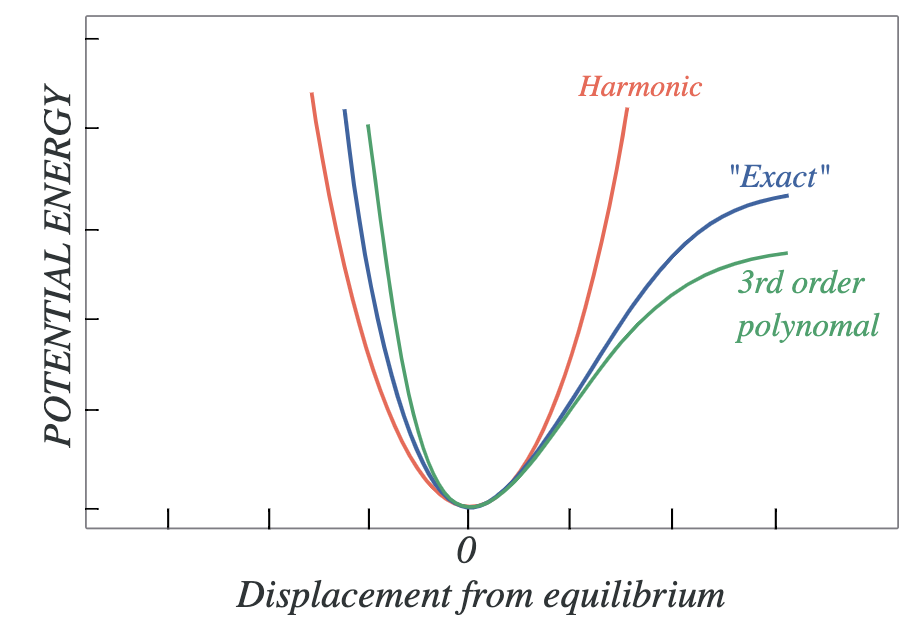
Fig. 62 Deviation from simple harmonic potential approximation (red curve) of true/exact potential (blue curve) with cubic term (green)#
Setting energy scale to be relative to \(U(x_0)=0\) and recongizing that first derivative vanishes at minima \(x_0\) we have
Hence we see that the Harmonic approximation is only the first non vanishing term! Furthermore we see that spring constant k and subsequent anharmonicity consnats such as \(\gamma\) are higher order derivatives of potential energy. That is the more non-linear the potential the higer the contribution of these terms. And vice verse clsoer the potential to quadratic form the more accurate is the harmonic assumtion.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
# Define the range for x values, focusing on the dissociation region
x = np.linspace(0, 2.5, 500)
# Define the harmonic potential (quadratic term)
harmonic = 0.5 * x**2
# Define the harmonic + cubic potential
harmonic_cubic = 0.5 * x**2 - 0.2 * x**3
# Define the harmonic + cubic + quartic potential
harmonic_cubic_quartic = 0.5 * x**2 - 0.2 * x**3 + 0.05 * x**4
# Define the harmonic + cubic + quartic + higher-order polynomial (5th and 6th order)
polynomial_approx = 0.5 * x**2 - 0.2 * x**3 + 0.05 * x**4 - 0.01 * x**5 + 0.001 * x**6
# Define the Morse potential
def morse_potential(x, D=1, a=1):
return D * (1 - np.exp(-a * x))**2
# Set parameters for Morse potential
D = 1 # Depth of the potential well
a = 1 # Width of the potential well
# Compute Morse potential
morse = morse_potential(x, D, a)
# Plot all the potentials, showing progression
plt.figure(figsize=(8, 6))
# Harmonic only
plt.plot(x, harmonic, label='Harmonic: $0.5 x^2$', color='b', lw=2)
# Harmonic + Cubic
plt.plot(x, harmonic_cubic, label='Harmonic + Cubic: $0.5 x^2 - 0.2 x^3$', color='g', lw=2)
# Harmonic + Cubic + Quartic
plt.plot(x, harmonic_cubic_quartic, label='Harmonic + Cubic + Quartic: $0.5 x^2 - 0.2 x^3 + 0.05 x^4$', color='r', lw=2)
# Polynomial approximation (up to 6th order)
plt.plot(x, polynomial_approx, label='Polynomial Approx (up to $x^6$)', color='c', lw=2)
# Morse potential
plt.plot(x, morse, label='Morse Potential', color='k', lw=3)
# Add labels and legend
plt.title('Polynomial Approximation of Harmonic Oscillator vs. Morse Potential')
plt.xlabel('x (dissociation region)')
plt.ylabel('Potential Energy')
plt.axhline(0, color='black',linewidth=0.5)
plt.axvline(0, color='black',linewidth=0.5)
plt.grid(True)
plt.legend()
plt.ylim([0, 2])
(0.0, 2.0)
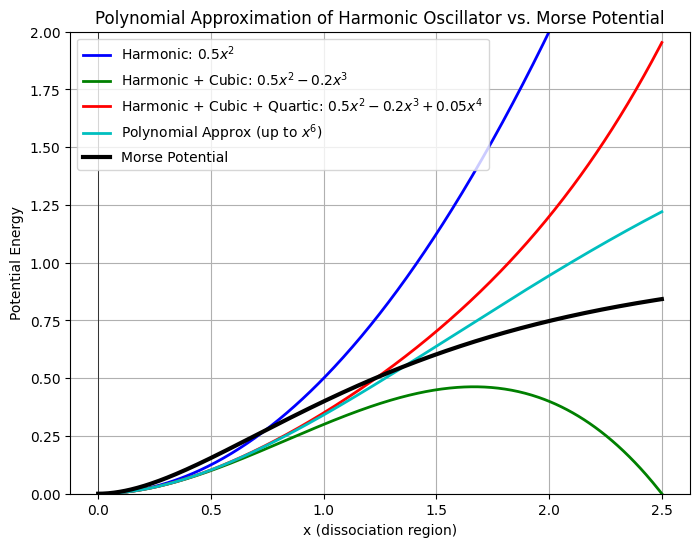